Verilog/SV elements
note
by default, the documenter will use //!
to recognize things to document.
Supported labels
Here are the following labels supported by the documenter:
- Module Description
- Ports
- Parameters
- Constants
- Registers / Wires
- Always Block
- Instances
- Functions
- Typedefs
Module
- Code
- Result
//! @title mymodule design
//! @author terosHDL
module mymodule #(
parameter PARAM1 = 1024 //! number of bytes in fifo
)(
output reg [PARAM1-1:0] data,
input clk, //! 300Mhz Clock
input rstn
);
endmodule
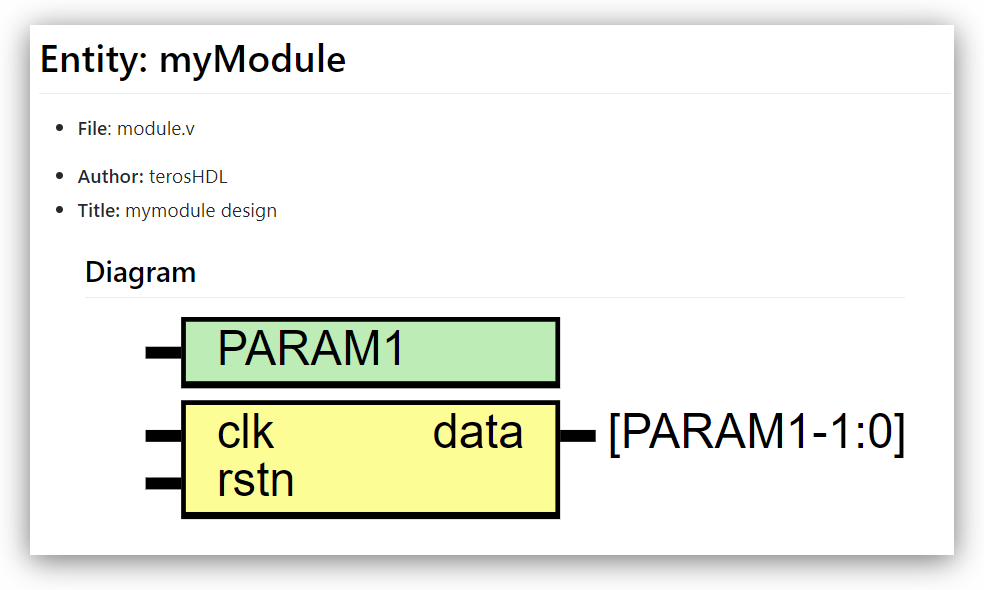
Ports
- Code
- Result
//! @title mymodule design
//! @author terosHDL
module mymodule #(
parameter PARAM1 = 1024 //! number of bytes in fifo
)(
output reg [PARAM1-1:0] data,
input clk, //! 300Mhz Clock
input rstn
);
endmodule
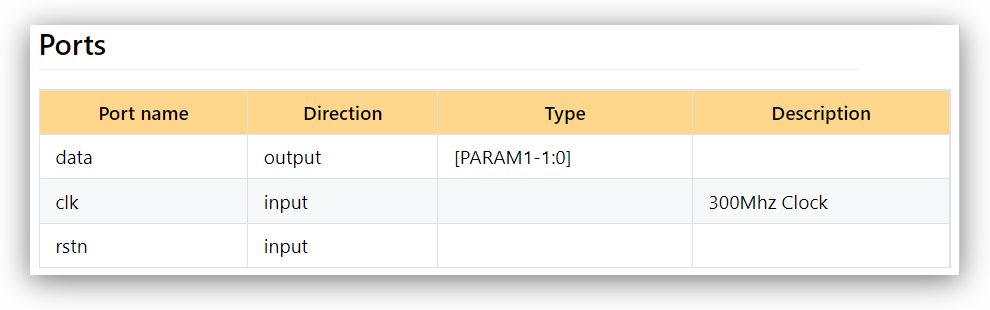
Parameters
- Code
- Result
//! @title mymodule design
//! @author terosHDL
module mymodule #(
parameter PARAM1 = 1024 //! number of bytes in fifo
)(
output reg [PARAM1-1:0] data,
input clk, //! 300Mhz Clock
input rstn
);
endmodule
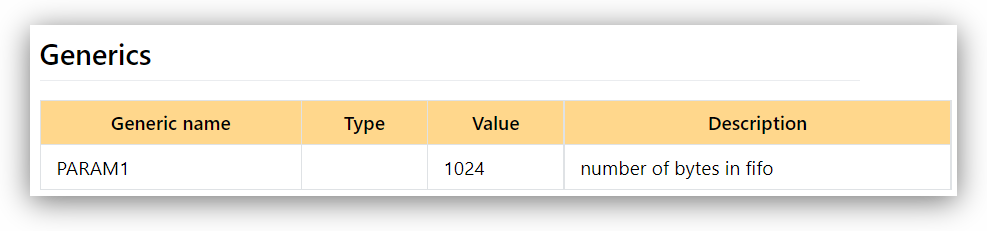
Constants
- Code
- Result
module myconsts ();
localparam SN=11223344; //! SN for this node
endmodule
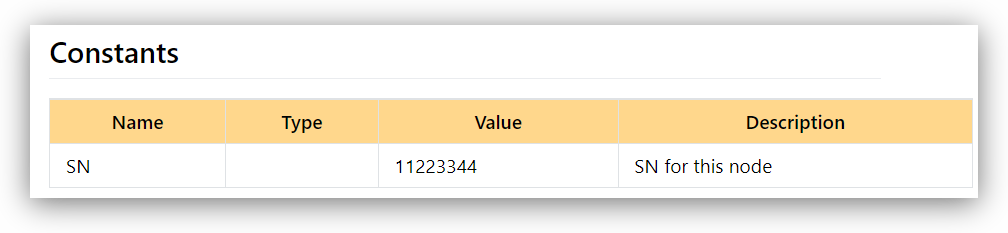
Always
- Code
- Result
module alwaysmod (
input clk,
input rstn
);
always @(posedge clk or negedge rstn) begin: myproc
end
endmodule
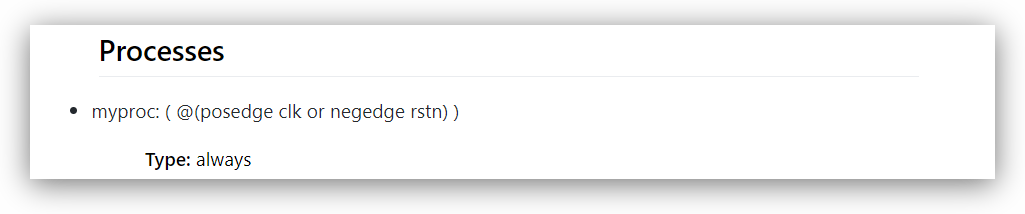
Instances
- Code
- Result
module tb_mytb(
input clk,
input rstn
);
mymodule dut(
.rstn (rstn),
.clk (clk)
);
endmodule
Functions
- Code
- Result
module funcs ;
function reg[1:0] myfunc(input a,b);
myfunc = {a,b};
endfunction
endmodule
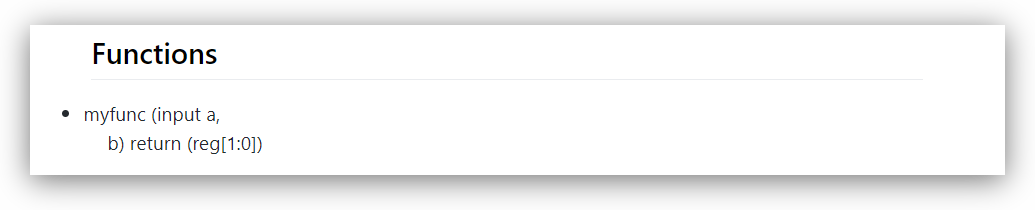
Typedefs
- Code
- Result
//! AXI-4 Stream
typedef struct packed {
logic [7:0] data;
logic [0:0] valid;
logic [0:0] clk;
} mystruct;
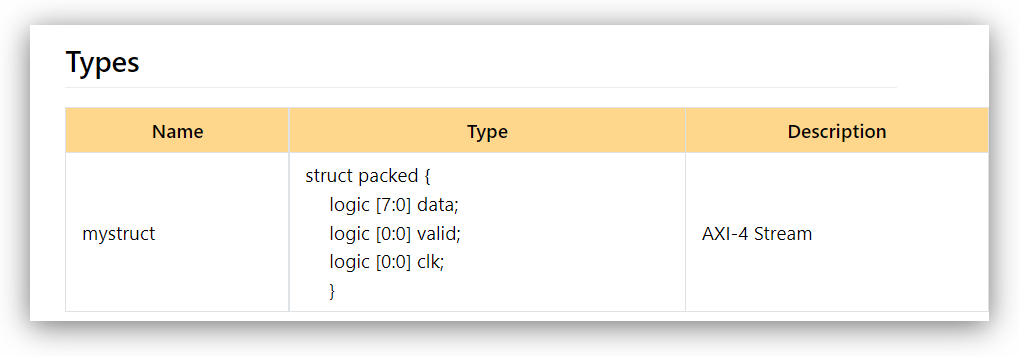