VHDL elements
note
by default, the documenter will use --!
to recognize things to document.
Supported labels
Here are the following labels supported by the documenter:
- Entity description
- Ports
- Generics
- Constants
- Signals
- Processes
- Instances
- Functions
- Types
- Type record
- Type enum
Entity
- Code
- Result
--! Entity example
-- description
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
use ieee.math_real.all;
entity myentity is
generic (
gen1 : integer := 0
);
port (
clk : in std_logic;
reset : in std_logic
);
end entity;
architecture rtl of myentity is
begin
end architecture;
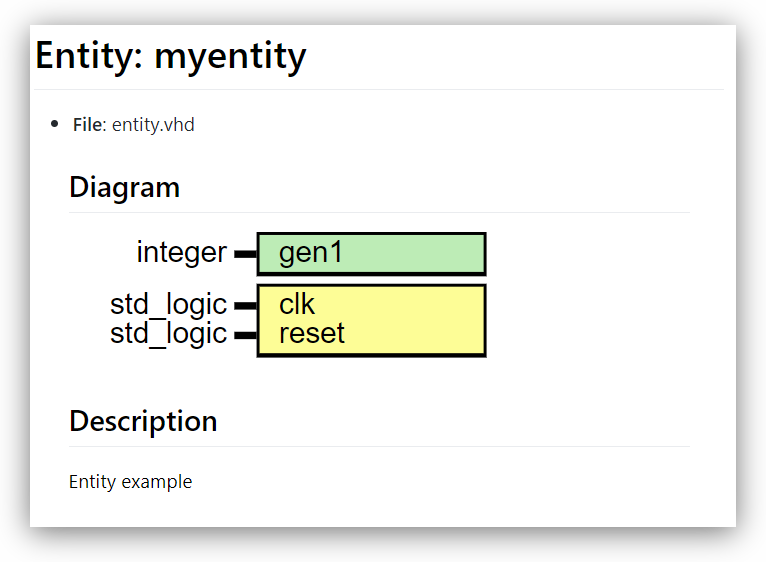
Package
- Code
- Result
package mynewpackage is
end package;
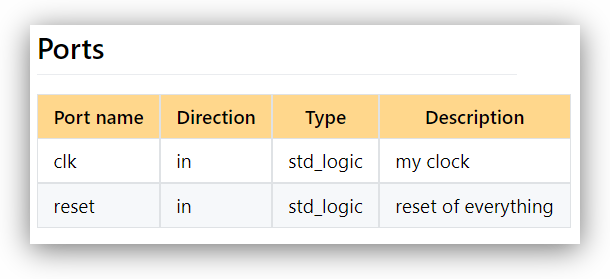
Generic
- Code
- Result
--! Entity example
-- description
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
use ieee.math_real.all;
entity myentity is
generic (
gen1 : integer := 0
);
port (
clk : in std_logic;
reset : in std_logic
);
end entity;
architecture rtl of myentity is
begin
end architecture;
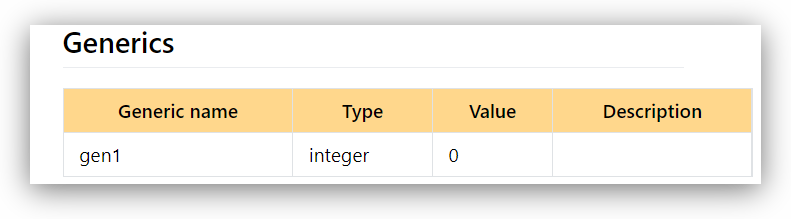
Port
- Code
- Result
entity ports is
port (
clk : in std_logic; --! my clock
reset : in std_logic --! reset of everything
);
end entity;
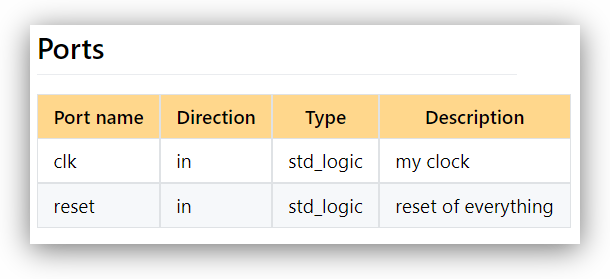
Signal
- Code
- Result
library ieee;
use ieee.std_logic_1164.all;
entity mysignal is
port (
clk : in std_logic;
reset : in std_logic
);
end entity mysignal;
architecture rtl of mysignal is
signal sig_a : std_logic_vector(31 downto 0);
signal sig_b : std_logic_vector(31 downto 0);
signal sig_c : std_logic; --! example documentation
signal data_a: integer range 0 to 1023;
begin
end architecture;
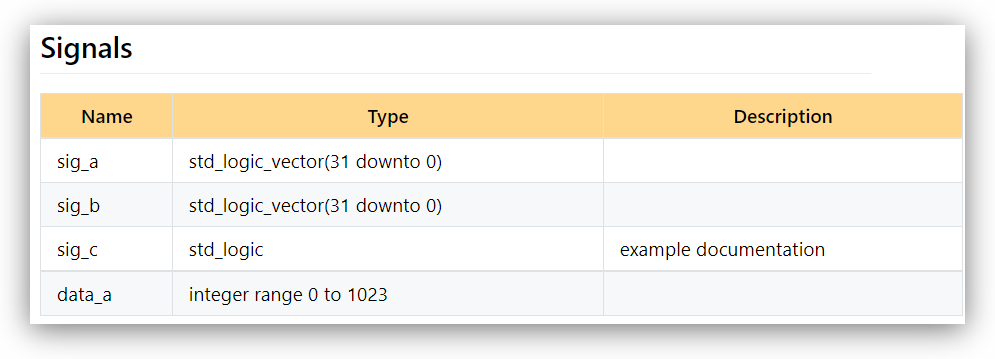
Constant
- Code
- Result
package mypackage is
constant DATA_WIDTH : integer := 35; --! number of bits
constant ADDR_WIDTH : integer := 21; --! number of bits
end package;
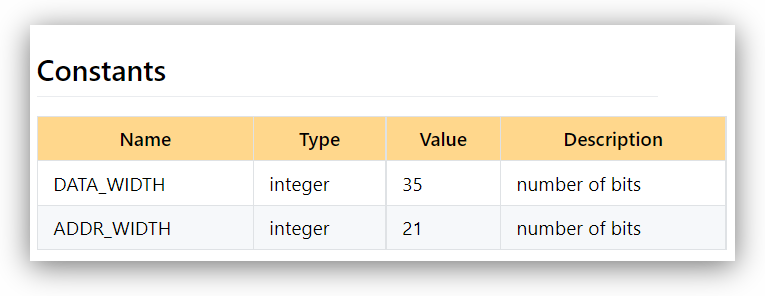
Function
- Code
- Result
library ieee;
use ieee.std_logic_1164.all;
package mypackage is
function func1 (x:integer) return std_logic;
end package;
package body mypackage is
function func1 (x:integer) return std_logic is
begin
end function;
end package body;
Process
- Code
- Result
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
use ieee.math_real.all;
entity my_process is
port (
clk : in std_logic;
reset : in std_logic
);
end entity my_process;
architecture rtl of my_process is
begin
myproc: process (clk)
begin
end process;
end architecture;
Type
- Code
- Result
library ieee;
use ieee.std_logic_1164.all;
package mytypes is
type t_my_custom_type is range 0 to 1000; --! my custom type description
end package;
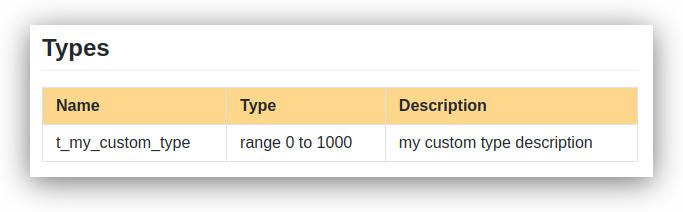
Type Record
- Code
- Result
library ieee;
use ieee.std_logic_1164.all;
package mytypes is
--! AXI Stream 32 bits
type t_axis_32b is record
tdata : std_logic_vector (31 downto 0); --! tdata description
tvalid : std_logic; --! tvalid description
tlast : std_logic; --! tlast description
tready : std_logic; --! tready description
end record t_axis_32b;
end package;
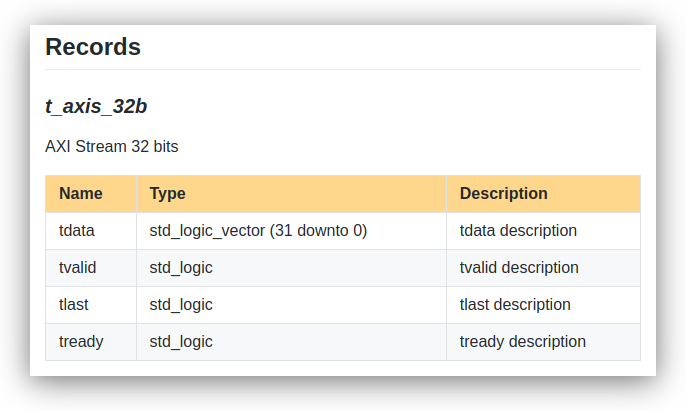
Type Enum
- Code
- Result
library ieee;
use ieee.std_logic_1164.all;
package mytypes is
--! main state machine description
type main_sm_type is (IDLE, --! IDLE state description
PUSHA, --! PUSHA state description
PUSHB, --! PUSHB state description
FINISH --! FINISH state description
);
end package;
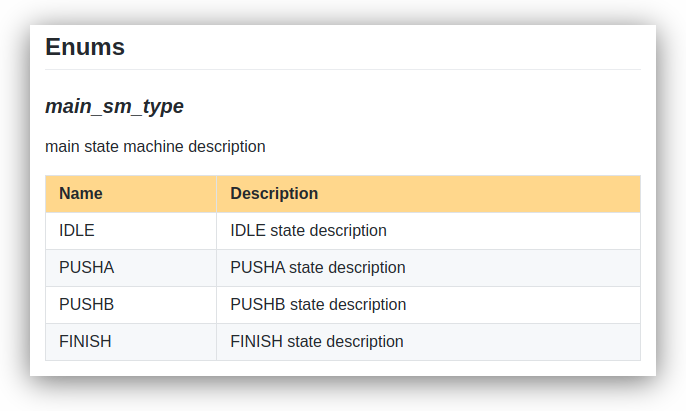